In today’s world, integrating AI into your applications can take user experience to the next level. One of the most powerful AI tools available is OpenAI’s ChatGPT, which can be used for a wide range of applications, from customer support bots to creative writing assistance. In this post, we will walk through how to Integrate ChatGPT API with PHP, enabling you to interact with the powerful AI directly from your web server.
What is ChatGPT?
ChatGPT is an advanced language model developed by OpenAI, capable of understanding and generating human-like text. Whether you’re building a chatbot, enhancing content creation, or automating responses, ChatGPT can be a valuable addition to your website or application. With the ability to handle conversational exchanges, it’s ideal for dynamic interaction in real-time.
Prerequisites
Before diving into the code, there are a few things you’ll need:
- OpenAI API Key: You must sign up for an OpenAI account and obtain an API key, which is required to interact with their services.
- PHP Setup: Ensure that PHP (7.2 or higher) is installed on your server, and that the cURL extension is enabled. cURL is necessary for making HTTP requests to the API.
- Basic Understanding of PHP: You should be comfortable working with PHP, understanding how to make API requests, and process JSON data.
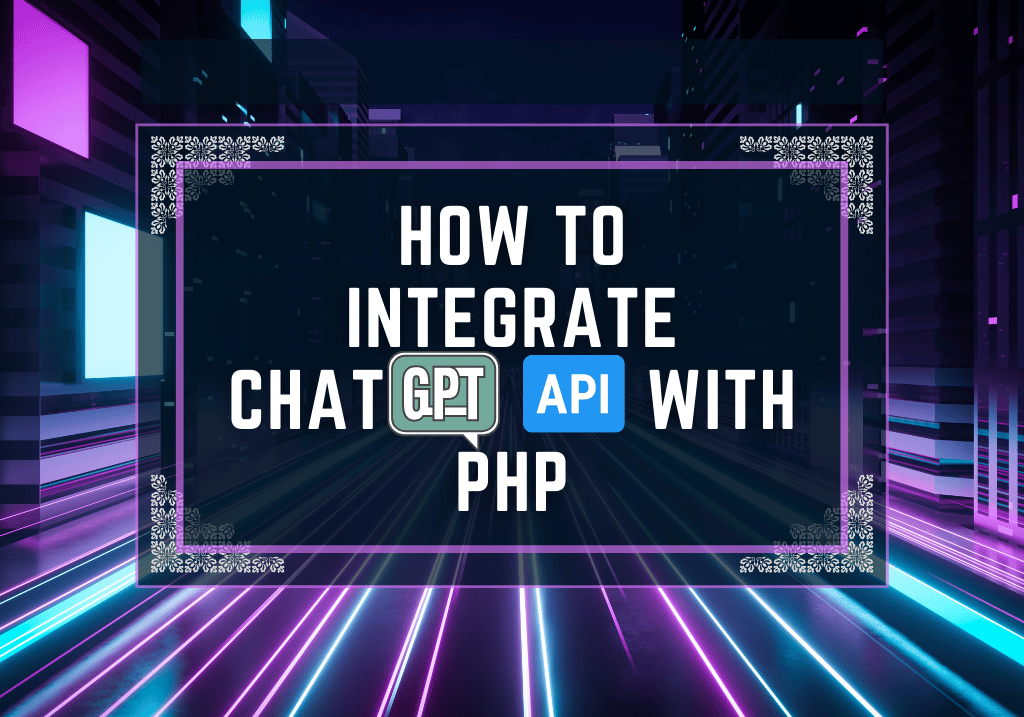
Step 1: Get Your OpenAI API Key
Head over to OpenAI’s platform and create an account if you haven’t already. After logging in, you’ll be able to generate an API key. Keep this key secure as it allows access to the OpenAI API.
Step 2: Set Up Your PHP Script
Create a PHP file that will handle communication with the OpenAI API. Below is a sample script to Integrate ChatGPT API with PHP:
<?php
// Your OpenAI API key (replace with your actual API key)
$apiKey = 'YOUR_API_KEY';
// The endpoint URL for OpenAI's ChatGPT API
$apiUrl = 'https://api.openai.com/v1/completions';
// Prepare the data to send in the request
$data = [
'model' => 'text-davinci-003', // You can change to the model you want to use, e.g., "gpt-3.5-turbo"
'prompt' => 'Say Hello, ChatGPT!', // The message you want to send to ChatGPT
'max_tokens' => 100, // The maximum number of tokens in the response
'temperature' => 0.7, // Control randomness (0.0 - 1.0)
];
// Encode the data to JSON format
$dataJson = json_encode($data);
// Initialize cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey,
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $dataJson);
// Execute the cURL request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Decode the response from JSON format
$responseData = json_decode($response, true);
// Output the response (you can modify this to use the response data as needed)
if (isset($responseData['choices'][0]['text'])) {
echo "ChatGPT: " . $responseData['choices'][0]['text'];
} else {
echo "Error: " . $responseData['error']['message'];
}
?>
Step 3: Customize and Test the Script
In the script above, there are several customizable elements:
- API Key: Replace
'YOUR_API_KEY'
with the API key you received from OpenAI. - Model: We’re using
text-davinci-003
, but OpenAI offers several models (e.g.,gpt-3.5-turbo
) with varying capabilities. Choose the one that suits your needs. - Prompt: This is the text you’ll send to the ChatGPT model. Change it to anything you want the AI to respond to.
- Max Tokens: This defines the maximum length of the response in tokens (where a token can be as short as one character or as long as one word). Adjust it based on how detailed you want the response to be.
- Temperature: This parameter influences the randomness of the response. Lower values (e.g., 0.2) make the responses more deterministic, while higher values (e.g., 0.8) encourage creative or unexpected responses.
Once you’ve set it up, you can run the script. If everything is configured correctly, you should see the response from ChatGPT printed out in your browser or command line.
Step 4: Handle Errors and Output
The script includes basic error handling. If the response from the API contains an error, it will be printed. You can extend this by adding more robust error management, such as logging the errors for troubleshooting.
Integrating ChatGPT into your PHP applications is a great way to enhance your website’s interactivity and functionality. By following these steps, you can start sending prompts to ChatGPT and receiving intelligent, conversational responses. With some further customization, you could Integrate ChatGPT API with PHP to automate tasks such as customer support, content generation, and more.
If you’re new to AI, this process will be an exciting introduction to exploring the possibilities of OpenAI’s models. Moreover, once you successfully Integrate ChatGPT API with PHP, you’ll be able to build more dynamic, engaging, and intelligent web applications that can respond to user queries in real-time.
Always remember to monitor your API usage, as OpenAI charges based on the number of tokens used in requests. Happy coding, and enjoy exploring the capabilities of AI.